Sometimes you will find a problem and not be able to work out the actual cause of the problem. No matter how much you debug or trawl through code and documentation you cannot find a reason for a bug. So you settle on a fix that works, but it’s really just hiding the problem.
The Observation
As I’ve been going through testing and fixing each version, I noticed a problem specific to the NeoForged 1.21.1 build. I couldn’t find any caterpillars, eggs, or chrysalises, anywhere in the world. It seemed that they were not spawning for some reason.
I didn’t have time to look at it right away, so I created an issue for it that I could come back to later. Obviously, there being a version where most of the entities don’t spawn is a big problem for a mod that adds new entities, so it wasn’t long before I came back to try and fix it.
I switched to the 1.21.1 branch and started testing. I first wanted to figure out why none of these entities were spawning, so I added some break points to the spawn code and started testing. As far as I could tell by breaking into the code, they were all spawning fine. I couldn’t find anything wrong when I ran the game.
Something else was going on. And luckily I spotted something that revealed the actual problem.
The Actual Problem(s)
I found a naturally spawned chrysalis. I could see its bounding box using F3+B
, but only if I got really close to it. If I moved more than 2 or 3 blocks away it would disappear. The problem is that these entities are being culled at too short a distance, making them impossible to spot from a distance.
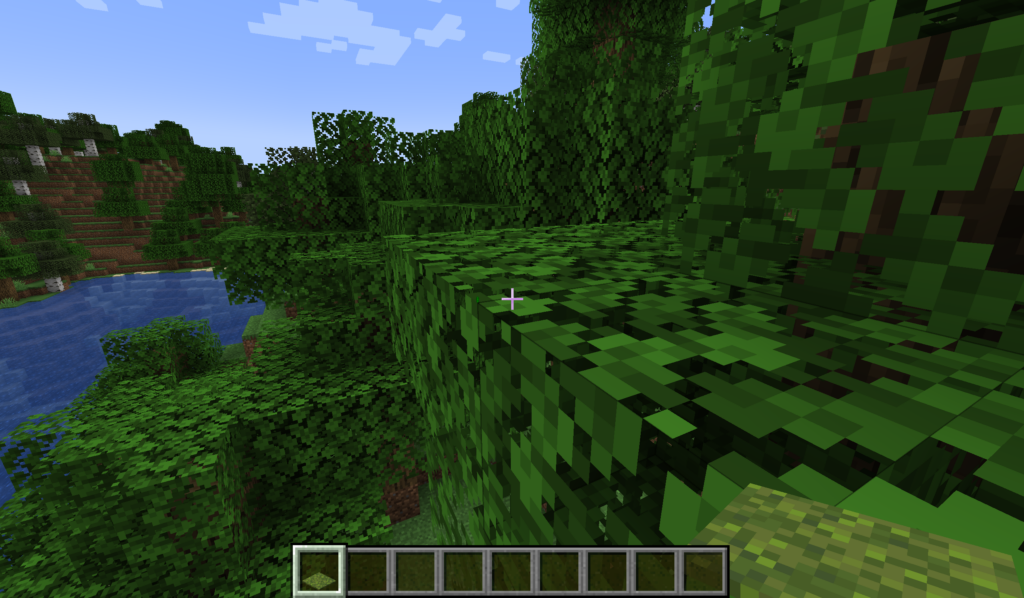
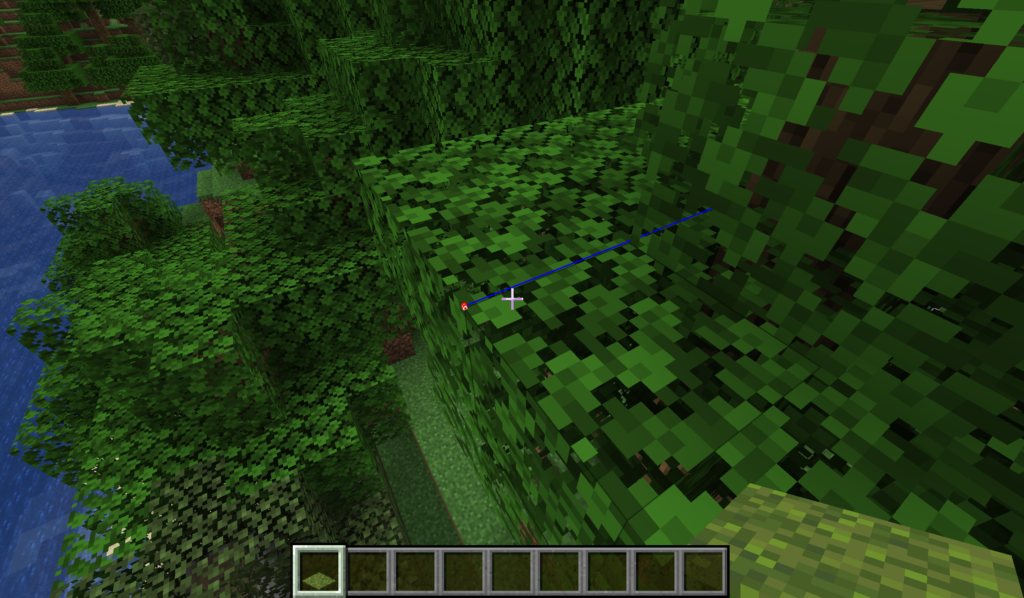
This wasn’t the only problem, however. The entities themselves were way too small. I did want these entities to be small, but in 1.21.1 they had been scaled down to the size of a pixel or two. I could see their bounding boxes, but I couldn’t see their models.
I didn’t know what was causing these size changes, but I needed to know if I wanted to fix this properly. Thus began the hunt.
Hunt for the Cause
My search didn’t get me any real answers. I could see from the primer that there had been changes to the renderer, but nothing in there stood out as affecting the size of entities. I broke into and searched through the code for any clues but found nothing.
I made a post to the Discord to see if anyone else might be able to weigh in. Unfortunately, this time would be in vain. I knew I could fix this bug, but I didn’t like the solution I had. But I had no other choice, this was the only way this was ever going to work.
The Cover-Up
The fix was a simple, and consisted of two parts. First, I would stop the entities from being culled so that players could see them from a distance. Second, I would simply multiply both the model sizes and the bounding boxes so they were a similar size to how they were with other versions of Minecraft.
Fixing the culling was easy. There is a shouldRenderAtSquareDistance
method in the Entity
class that I could override in order to ensure the entities render. By default the distance an entity can be seen is scaled to its size, but in my version the smallest limit will always be 64 blocks:
/** * Overridden so that butterfly entities will render at a decent distance. * @param distance The distance to check. * @return TRUE if we should render the entity. */ @Override public boolean shouldRenderAtSqrDistance(double distance) { double d0 = this.getBoundingBox().getSize(); if (d0 < 1.0D) { d0 = 1.0D; } d0 *= 64.0D * getViewScale(); return distance < d0 * d0; }
Now the entities would render, but they would always be way too small. I needed to increase the size of both the models, and the bounding boxes. With some experimentation I realised that butterflies and smaller entities would need different values, so I created two constants in the butterfly data to use:
public static final float BUTTERFLY_SIZE_MOD = 1.25f; public static final float DIRECTIONAL_SIZE_MOD = 16.0f;
I could then use these values to update the scale at which they were rendered:
/** * Scale the entity down. * @param entity The entity. * @param poses The current entity pose. * @param scale The scale that should be applied. */ @Override protected void scale(@NotNull ButterflyEgg entity, PoseStack poses, float scale) { float s = entity.getScale() * ButterflyData.DIRECTIONAL_SIZE_MOD; poses.scale(s, s, s); }
And I could also update the size of their bounding boxes when they’re registered:
/** * Register the caterpillars. * @param butterflyIndex The index of the caterpillar to register. * @return The new registry object. */ private DeferredHolder<EntityType<?>, EntityType<Caterpillar>> registerCaterpillar(int butterflyIndex) { float sized = 0.1f * ButterflyData.DIRECTIONAL_SIZE_MOD; return this.deferredRegister.register(Caterpillar.getRegistryId(butterflyIndex), () -> EntityType.Builder.of(Caterpillar::new, MobCategory.CREATURE) .sized(sized, sized) .build(Caterpillar.getRegistryId(butterflyIndex))); }
This fix worked. The entities were now visible and rendered at sizes that seemed consistent with other versions.
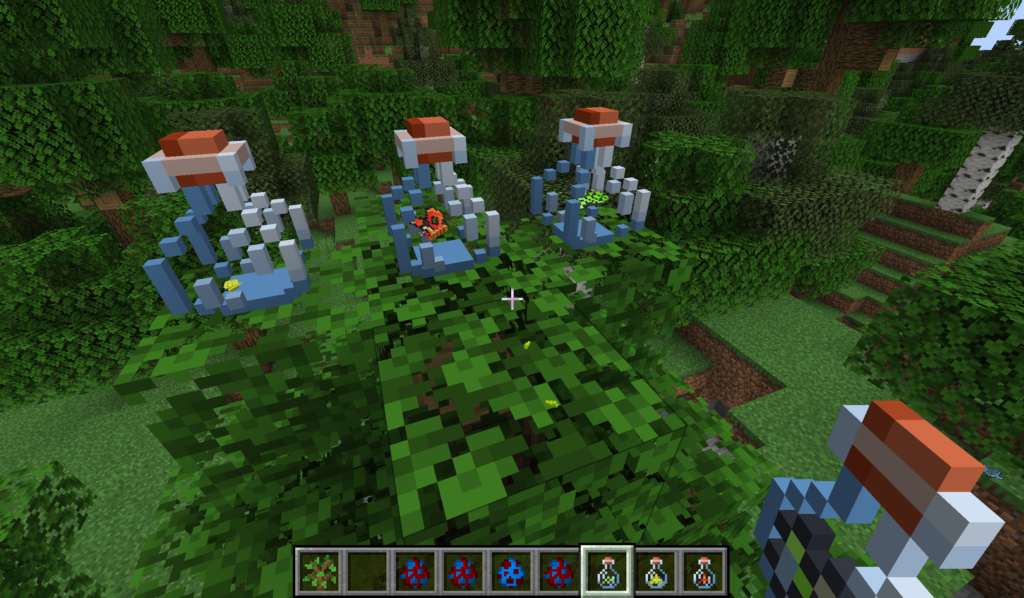
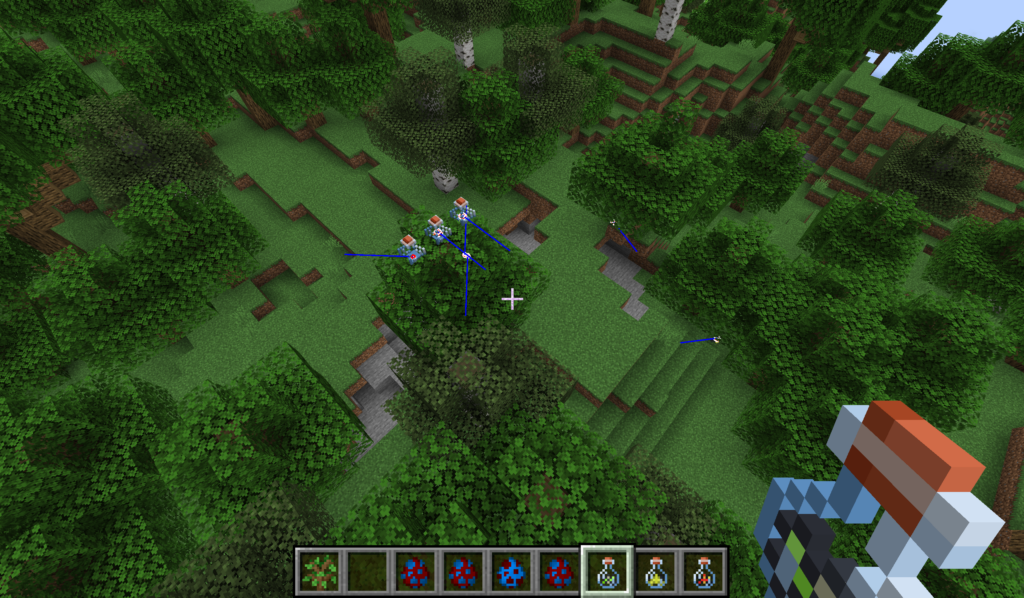
But I didn’t like the fix. It didn’t address the root cause. Like painting over rotting wood, it makes it look nice but the rot is still there. It bugged me that I didn’t find the cause, and that I could not find a real fix.
A Lucky Break
I wasn’t quite ready to give up. I kept searching and going through documentation hoping to figure this out. Then I found it in the NeoForge primer for 1.20.5:
net.minecraft.world.entity.LivingEntity
getScale
->getAgeScale
getScale
still exists and handles scaling based on an attribute property
I was using a method named getScale
, but it wasn’t meant to be overriding any of the base entity behaviour. But if LivingEntity
also has this method, then I was inadvertently changing anything else that used this method.
It all clicked into place. There had been a lot of changes to the renderer in 1.21.1, and many of those changes may have involved this method. That’s why everything seemed to scale and cull in such odd ways. And now I knew why it was happening, I knew how to fix it.
A Real Fix
I renamed getScale
to getRenderScale
. I did this for all versions, and now the size of all entities is consistent across all versions. This fix is not only simpler, but it actually removes the rot at the core, so there are no more bugs due to scaling. And by applying this fix to all versions, they all have parity and will behave the same when it comes to entity sizes.
This bug was a particularly hard one to figure out, even though I knew a way to get around the problem. I thought it was a problem with a single version, but it turned out to be a problem across all versions of the mod.
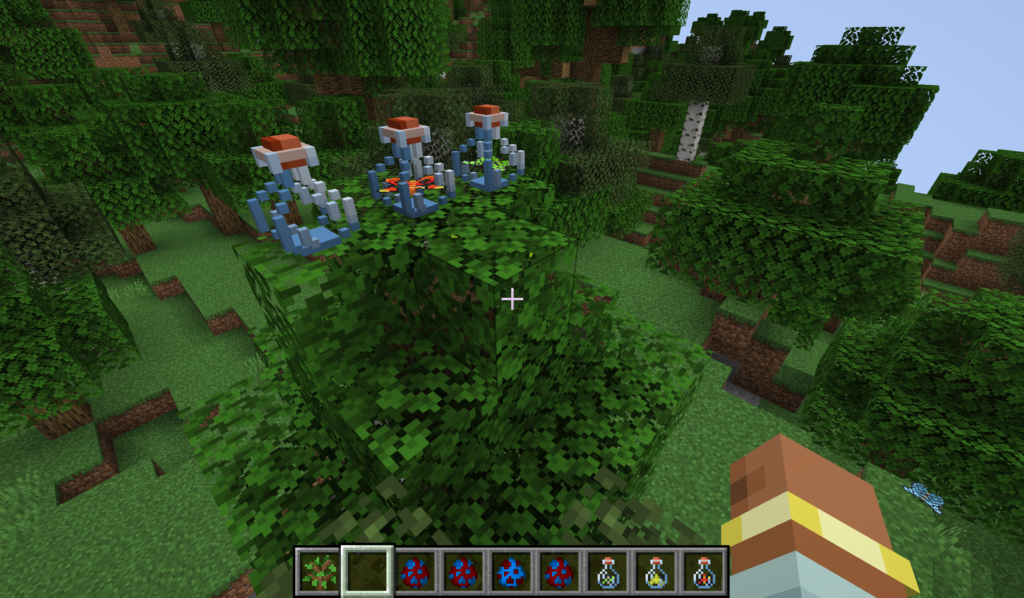
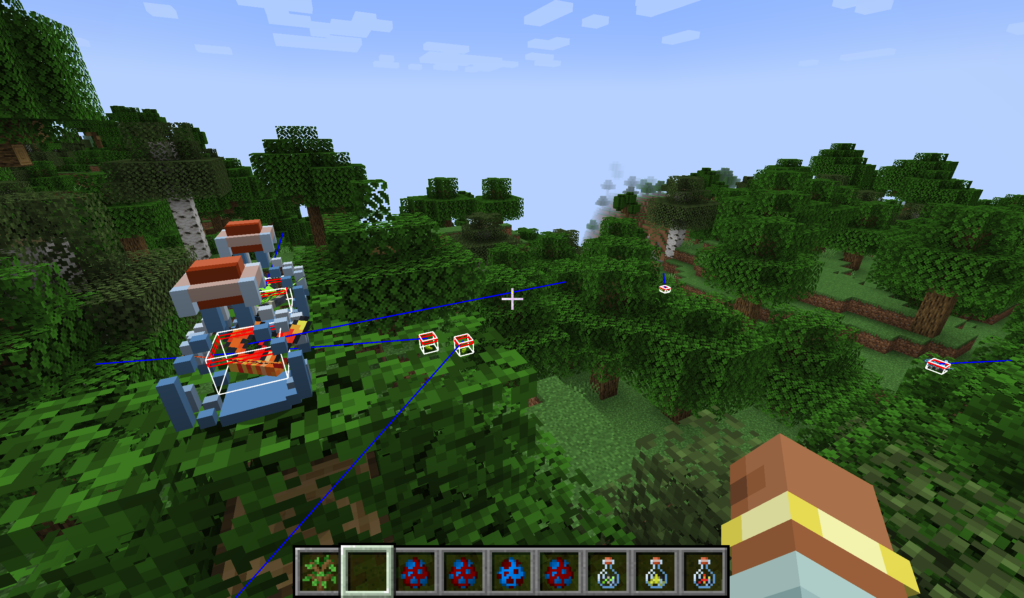
I knew that I needed to find out why it was happening, and my persistence paid off. If I hadn’t managed to find this fix, then even more problems could have cropped up later in development. Instead of covering up a problem which would only reappear later, I know understand the problem and have a real fix for it.