This week I spent some time fixing a few bugs I’ve been noticing while testing the mod. Now our butterflies look better, animate better, and spawn better. These things may seem minor in comparison to adding cool new features, but as I’ve said before: details matter.
Caterpillar Spawning
The first bug that I noticed was that caterpillars would often drop from their block when spawning. After a bit of testing, I found that it was failing when caterpillars spawned on 3 sides of a block: UP, SOUTH, and EAST. The positive directions.
I broke into the code and found that the surface block would be incorrect for these directions. The fix was to check the direction of the creature and get a different block depending on the direction.
/** * Get the position of the block the caterpillar is crawling on. * @return The position of the block. */ protected BlockPos getSurfaceBlockPos() { return switch (this.getSurfaceDirection().getAxisDirection()) { case POSITIVE -> this.blockPosition(); case NEGATIVE -> this.blockPosition().relative(this.getSurfaceDirection()); }; }
After testing this code I found that caterpillars would now use the correct surface block to determine how it should move and if it should fall.
Butterfly Model
There have been a few problems with the butterfly model for a while, but every time I’ve tried to fix it I’ve struggled. This week I finally decided to just recreate the model from scratch using Blockbench, a tool that is designed to help create models for Minecraft. You can export the model to Java code, and then use it in your mod.
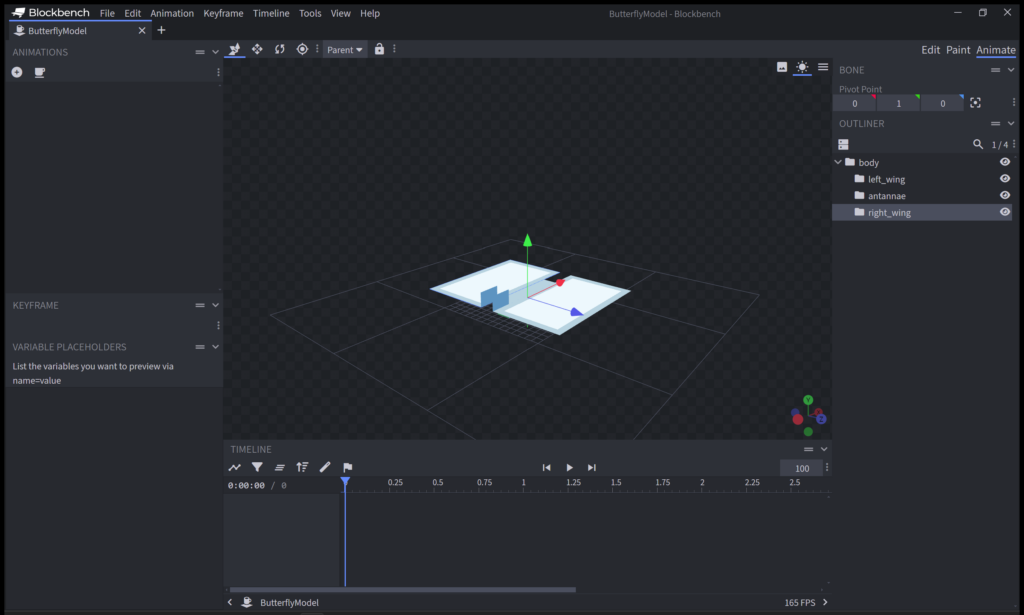
Using this tool I’m also able to set pivot points for specific parts of the model. These control the point which the part will rotate around, which is especially useful for making the wings rotate properly. One thing I don’t like about the mod at present is how landed butterflies look, but with the new model the wings line up much nicer.
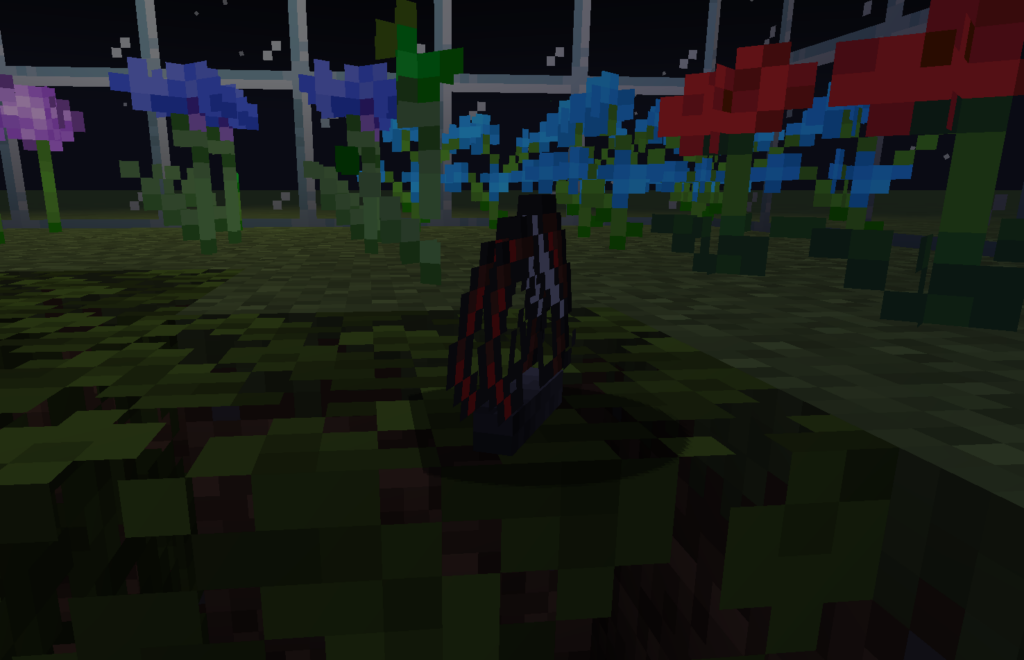
I was also able to import my butterfly textures into Blockbench so I could get a preview of what they will look like in-game. By doing this I spotted a few errors in some of the textures and was able to fix them.
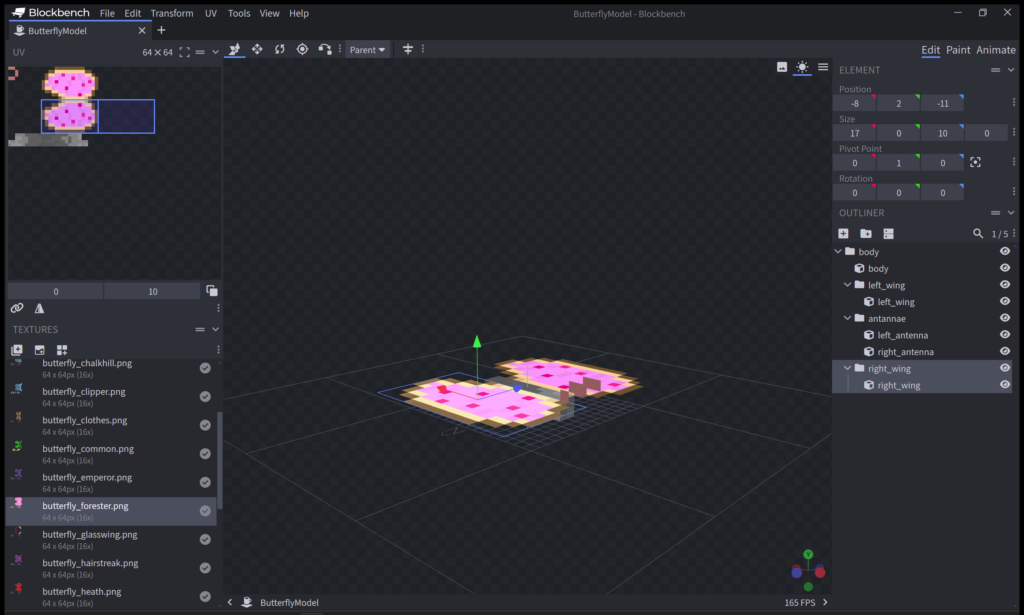
I also made a few tweaks here and there, overall improving the animation of butterflies and moths as they land.
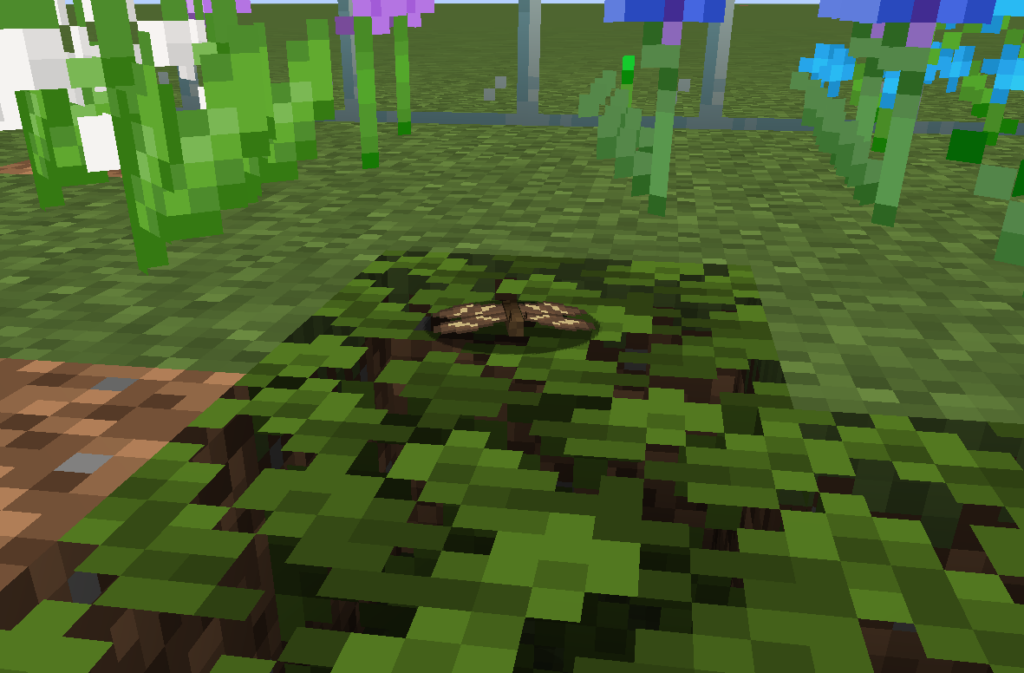
Saving the Landing
Another bug I noticed during my testing was that the Landed state of a butterfly wasn’t saved when you reloaded a world. They would look like they have landed, but on a reload they would be flapping their wings as if they were flying.
The solution here was to remove all calls that set the landed state to false
, apart from the stop()
methods of the egg-laying and resting goals. The game will put them back into the same goal and load the landed state from the save data, so we don’t need to override it unless they exit either of these goals.
Now we can leave a world and come back to butterflies that still keep their wings still when they are resting.
Luna Moth
I didn’t want to go another week without adding a new moth, so I created the Luna Moth this week. The moth is absolutely beautiful in real life, and I tried to reflect that in my art. I don’t think I did it justice but, it’s still looking pretty good.
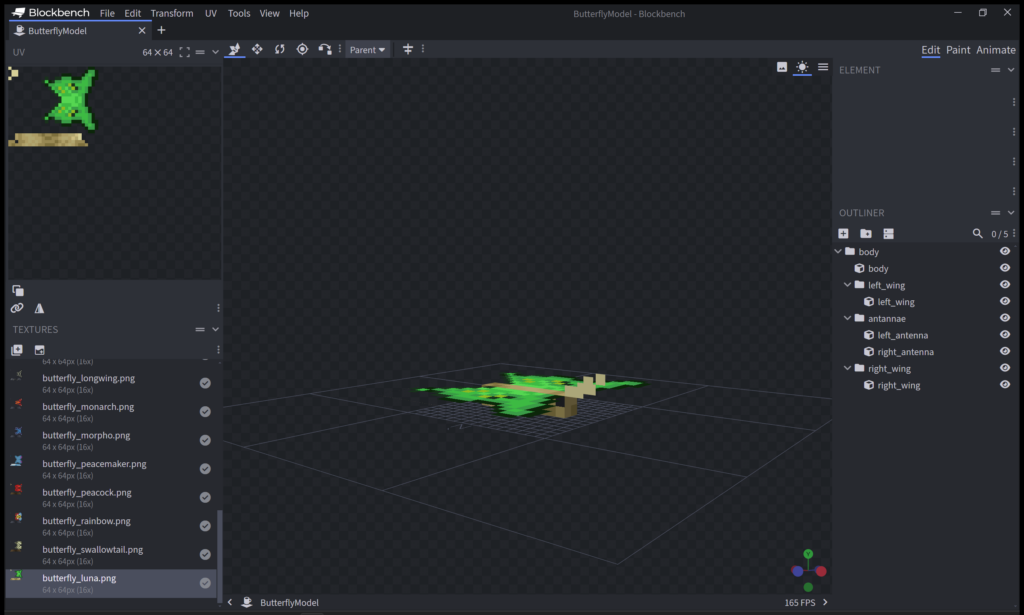
This moth is a little different in that it’s caterpillar will make clicking noises when a player gets nearby. I realised at this point that I haven’t added a single sound to the mod, so this is something new. I’m not a recording artist, so I need to source a sound from somewhere. I found something that sounded close to what I wanted on Freesound by LucasDuff.
The sound effect is under Creative Commons, so I’m free to modify it. I used Audacity to speed up the sound and alter the pitch a little until it sounded more like what I wanted. It’s not realistic, but it will let players know there is a luna moth caterpillar in the area.
To add the sound to Minecraft we need to have a sounds.json
file in our assets\butterflies
folder. This file defines all the sounds in the mod, alongside their subtitles.
{ "luna": { "subtitle": "butterflies.subtitle.luna_caterpillar", "sounds": [ "butterflies:luna_caterpillar" ] } }
Playing the sound is easy. I removed an override of getSoundVolume()
that muted the caterpillars, and added a new override for their ambient sounds.
/** * Return an ambient sound for the caterpillar. If the sound doesn't exist * it just won't play. * @return A reference to the ambient sound. */ @Nullable @Override protected SoundEvent getAmbientSound() { return SoundEvent.createVariableRangeEvent(new ResourceLocation(ButterfliesMod.MODID, ButterflyData.getSpeciesString(this))); }
By using getSpeciesString()
here I give myself the option of adding sounds to more caterpillars later. If the sound doesn’t exist, Minecraft simply ignores it, so we don’t need to worry about it.
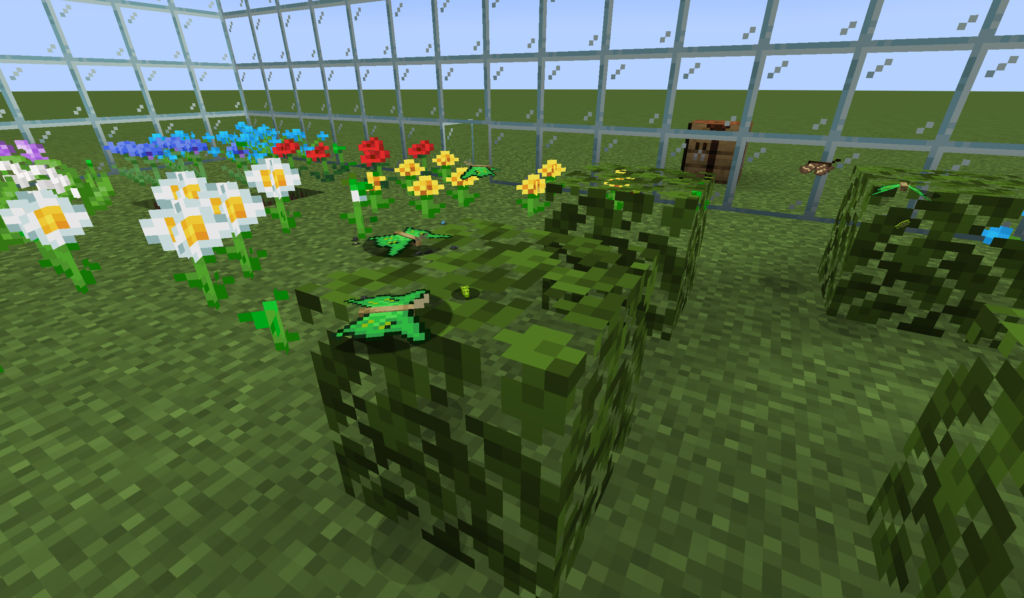
Two moths down. Only several more to go!